参考网址:
AtsushiSakai/PythonRobotics: Python sample codes for robotics algorithms. (github.com)
基于dijkstra算法,实现路径规划
机器人路径规划、轨迹优化系列课程_哔哩哔哩_bilibili
AStarSearch
算法基本思想
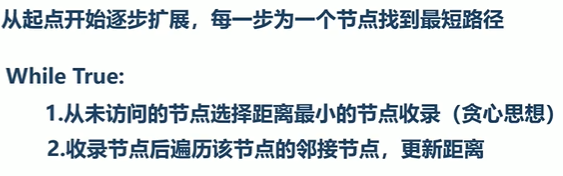
代码流程
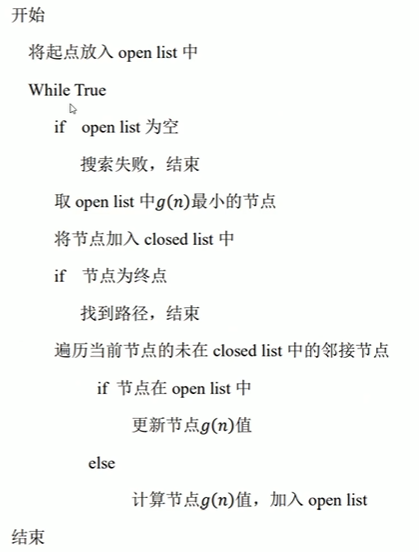
使用优先级队列实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| fill(dist, dist + nV + 1, INF);
priority_queue<P, vector<P>, greater<P> > q; dist[s] = 0;
q.push(P(0, s)); while (!q.empty()) { P p = q.top(); q.pop(); int v = p.second; if (dist[v] < p.first) continue; if (v == end) break; for (int unsigned i = 0; i < G[v].size(); i++){ Edge &e = G[v][i]; int dis = dist[v] + e.cost; if (dist[e.to] > dis){ dist[e.to] = dist[v] + e.cost; q.push(P(dist[e.to], e.to)); G4[v].push_back(e); } else if (dist[e.to] == dis){ G4[v].push_back(e); } } }
|
Astar算法
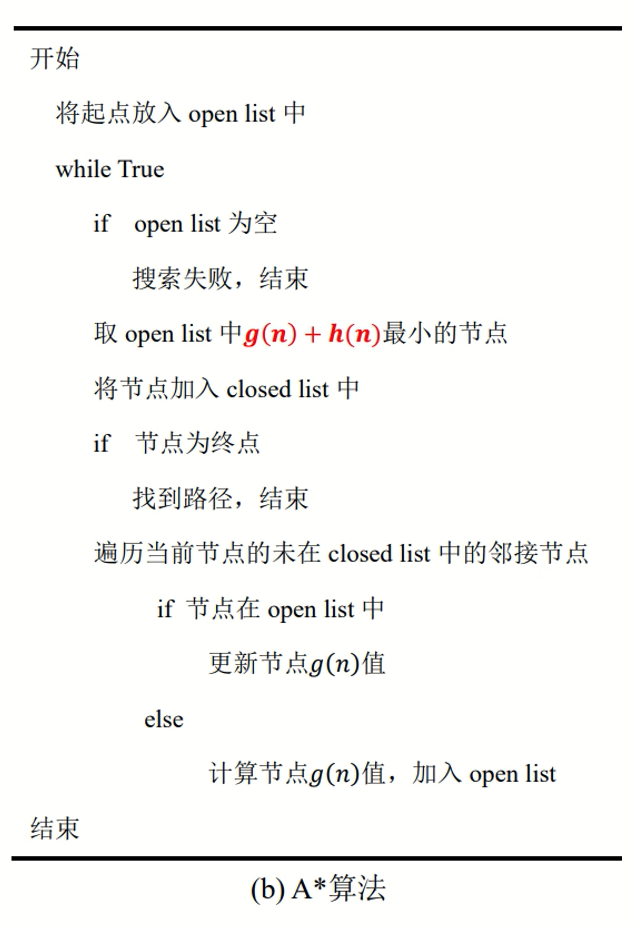
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76
| APoint* CAstar::findWay(APoint *beginPoint, APoint *endPoint,vector< vector<APoint*> >& allPoints) { _allPoints = allPoints; _endPoint = endPoint;
if (_endPoint->type == AType::ATYPE_BARRIER) { cout<<"终点是障碍"<<endl; return nullptr; } if (*_endPoint == *beginPoint) { cout<<"起始点相同"<<endl; return nullptr; } _openList.push_back(beginPoint); beginPoint->type = AType::ATYPE_OPENED; beginPoint->f = getF(beginPoint); do { _curPoint = _openList[0]; _openList.erase(_openList.begin()); _curPoint->type = AType::ATYPE_CLOSED; _closeList.push_back(_curPoint); if (*_curPoint == *_endPoint) { cout<<"have find way"<<endl; return _curPoint; } vector<APoint*> neVec = getNeighboringPoint(_curPoint); for (int i = 0; i<neVec.size(); i++) { auto tmpoint = neVec[i]; if (tmpoint->type == AType::ATYPE_CLOSED) { continue; } if (tmpoint->type != AType::ATYPE_OPENED) { tmpoint->parent = _curPoint; tmpoint->g = _curPoint->g + 10; tmpoint->h = getH(tmpoint); _openList.push_back(tmpoint); tmpoint->type = AType::ATYPE_OPENED; } else { if (tmpoint->h < _curPoint->h) { tmpoint->parent = _curPoint; tmpoint->g = _curPoint->g + 10; } } } sort(_openList.begin(), _openList.end(), mySort); } while (_openList.size()>0); cout<<"---can not find way---"<<endl; return nullptr; }
|